🚀 React: A Revolution in Web Development 🚀
- himubuss2910
- Oct 14, 2023
- 9 min read
Introduction: In the fast-paced realm of web development, React has emerged as a transformative and game-changing library. Developed by Facebook and nourished by a thriving community of enthusiasts and corporations, React, also known as React.js or ReactJS, has ushered in a new era of web application development. This introduction section provides an in-depth understanding of React's origins, its significance, and the core principles that underpin its approach to building user interfaces.
The Birth of React: React was born within the confines of Facebook. As Facebook's front-end application grew in complexity, it became clear that there was a need for a better way to manage and maintain the user interface. This realization led to the creation of React in 2011 by software engineer Jordan Walke. While React initially served Facebook's internal requirements, its potential quickly became apparent to the broader development community.
Community-Driven Excellence: React's journey has been anything but solitary. It has been embraced by a community of developers and organizations who recognize its potential. The open-source nature of React means that it's constantly evolving, improving, and staying at the forefront of web development technology. The library's governance and development process actively involve contributors worldwide, ensuring it remains robust and aligned with industry standards.
React's Pioneering Philosophy: React is built upon a set of fundamental principles that make it a standout choice for building web interfaces:
Declarative UI: React introduces a declarative approach to user interface development. Rather than imperatively defining how the UI should change with each update, you declare what the UI should look like for any given state. React takes care of the rest, efficiently updating the UI as needed.
Component-Based Architecture: At the heart of React lies the concept of components. These self-contained, reusable building blocks provide a modular structure to your application. Think of components as LEGO pieces you can assemble into intricate structures.
Virtual DOM: One of React's most powerful features is its use of the Virtual DOM. Instead of directly manipulating the real Document Object Model (DOM), React uses a virtual representation. This virtual DOM significantly enhances performance by minimizing direct interaction with the actual DOM.
JSX (JavaScript XML): React employs JSX, a syntax extension that enables developers to write HTML-like code within JavaScript. This approach simplifies the definition of your application's structure and layout, making your code more readable and maintainable.
Unidirectional Data Flow: React enforces a unidirectional data flow, ensuring that data moves in a single direction, typically from parent components to child components. This unidirectional nature leads to a predictable and easily debuggable application state.
React's philosophies have struck a chord with developers and have been instrumental in its rise to prominence. In the following pages, we will explore React's features in greater detail, its real-world applications, its inner workings, and its influence on modern web development.
If you would like me to expand on any specific aspect or have any particular questions about React's introduction, feel free to ask.
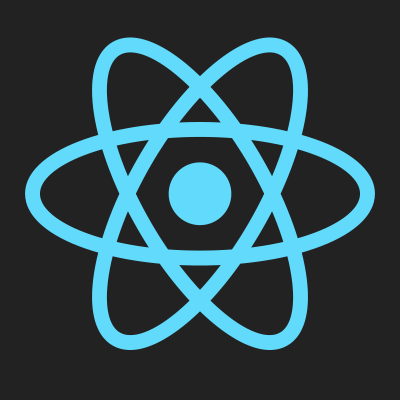
🌐 React's Versatility in Real-World Applications 🌐
Introduction: React is not merely a library confined to the realms of web development theory. It is a robust and versatile tool that powers numerous real-world applications across various industries. In this section, we'll explore some of the most prominent applications of React, uncovering how it's transforming the digital landscape.
1. Web Applications: React has established itself as a leading choice for developing web applications. Its component-based architecture allows developers to create intricate, interactive web interfaces with ease. Platforms like Facebook, Instagram, Airbnb, and WhatsApp have harnessed React's potential to build stunning user interfaces.
2. Mobile Applications: React Native, an offshoot of React, is designed specifically for mobile app development. React Native enables developers to create native mobile applications for both iOS and Android using a single codebase. This approach significantly reduces development time and maintenance effort. Popular apps like Facebook, Instagram, and Airbnb are powered by React Native.
3. E-Commerce Platforms: E-commerce companies leverage React to craft seamless, user-friendly online shopping experiences. The ability to build interactive product catalogs, shopping carts, and dynamic user interfaces makes React a preferred choice for industry giants like Amazon, Walmart, and Shopify.
4. Social Media Networks: Social media platforms thrive on engaging user interfaces, and React has revolutionized this landscape. With features like real-time updates and interactive posts, React has contributed to the success of social networks such as Twitter, Pinterest, and TikTok.
5. Content Streaming Services: React's capabilities extend to content streaming services. It helps provide users with a smooth, visually pleasing experience when browsing, searching, and streaming content. Platforms like Netflix and Hulu employ React for their web applications.
6. Online Gaming: Online gaming platforms rely on React to build captivating gaming interfaces. React's dynamic nature makes it an ideal choice for rendering game elements, managing player interactions, and facilitating real-time gaming experiences.
7. Financial Services: Even in the financial sector, React shines. Banks, investment firms, and financial institutions harness React to create secure, interactive web applications for tasks such as online banking, trading, and portfolio management.
8. Education and E-Learning: In the world of education, React is used to craft user-friendly, interactive e-learning platforms. Students and educators benefit from rich content, real-time collaboration, and immersive learning experiences.
9. Healthcare and Telemedicine: React contributes to the development of healthcare applications, including telemedicine platforms that allow patients to connect with healthcare providers remotely. These applications provide secure communication, appointment scheduling, and health record management.
10. Travel and Booking Services: Travel and booking companies rely on React for their platforms. Features like interactive maps, real-time booking, and personalized recommendations enhance the user experience on websites like Airbnb, Expedia, and Booking.com.
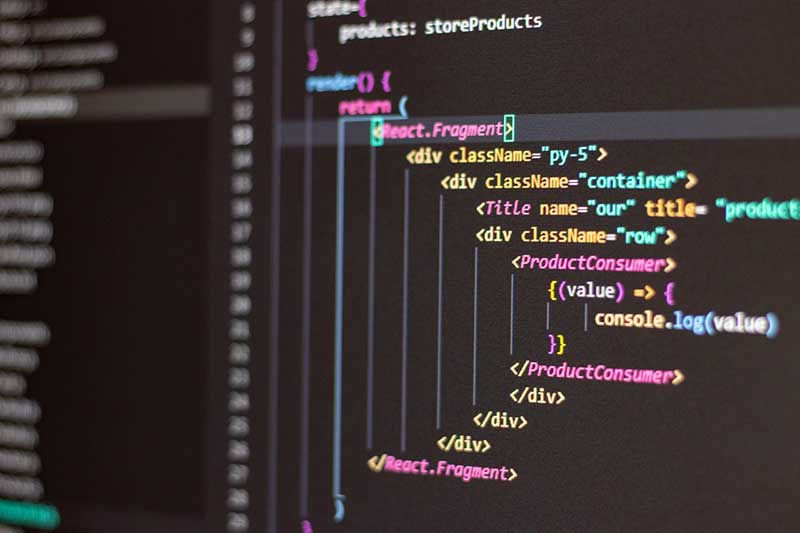
🧰 Building Blocks of React 🧰
React is built on a set of core concepts and principles that make it a powerful and efficient library for building user interfaces. In this section, we will explore these fundamental ideas, which serve as the building blocks of React development.
1. Components:
Definition: Components are the building blocks of React applications. They are reusable, self-contained pieces of user interface, like buttons, forms, or entire sections of a web page.
Use Case: Components make your code more organized, maintainable, and scalable. They can be customized, combined, and nested to create complex UIs.
2. Virtual DOM:
Definition: The Virtual DOM is a lightweight copy of the actual DOM (Document Object Model). React uses it to optimize and speed up rendering.
Use Case: When a change occurs in your application, React first updates the Virtual DOM and then calculates the most efficient way to update the real DOM. This process significantly improves performance.
3. JSX (JavaScript XML):
Definition: JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript files.
Use Case: JSX simplifies the creation of React elements, making your code more readable and maintainable. It lets you describe the UI components' structure and appearance.
4. State and Props:
Definition: State represents the data specific to a component, while props (short for properties) are inputs that are passed to a component.
Use Case: Components can change their state, which triggers re-rendering. Props enable the passing of data and behavior between components, facilitating the composition of complex UIs.
5. Component Lifecycle:
Definition: Components in React have a lifecycle that consists of several phases, such as creation, updating, and destruction.
Use Case: Understanding the component lifecycle is crucial for managing side effects, fetching data, and optimizing performance.
6. Hooks:
Definition: Hooks are functions that allow you to "hook into" state and lifecycle features from functional components.
Use Case: They simplify the development of functional components by enabling them to manage state, side effects, and more, previously only possible with class components.
7. Conditional Rendering:
Definition: React allows components to render content conditionally based on a set of conditions.
Use Case: Conditional rendering is essential for creating dynamic user interfaces that adapt to user interactions or data changes.
8. Event Handling:
Definition: React provides a straightforward way to handle user interactions, such as clicks, keyboard input, or touch events.
Use Case: Event handling allows you to add interactivity to your components, making them respond to user actions.
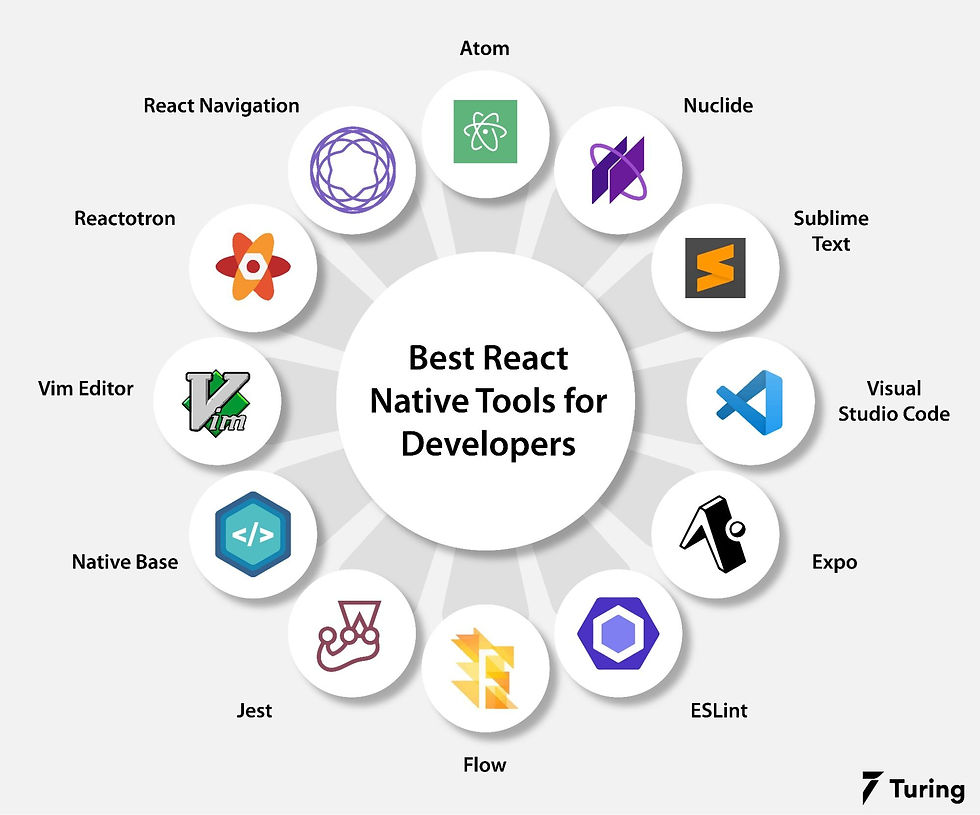
🛠️ Tools for Developing with React 🛠️
In the world of web development, having the right tools at your disposal can make all the difference. React is no exception, and there is a rich ecosystem of tools and libraries that complement React's capabilities. In this section, we'll explore some of the most popular tools and discuss how they enhance the development experience.
1. Create React App:
Description: Create React App is an officially supported tool for bootstrapping React applications. It sets up your project with a sensible default configuration, so you can start coding right away.
Benefits: It saves time by eliminating the need to configure your build tools and dependencies manually. You can focus on writing code instead of spending hours on setup.
2. React Router:
Description: React Router is a library for handling routing in React applications. It provides components and configuration for creating navigation within a single-page application.
Benefits: Routing is essential for creating multi-page experiences in single-page apps. React Router simplifies the process and helps maintain a clean URL structure.
3. Redux:
Description: Redux is a state management library for managing the state of your application. It follows a predictable and unidirectional data flow pattern.
Benefits: For complex applications, managing state can become challenging. Redux helps you organize and centralize your application's data and logic.
4. Axios:
Description: Axios is a popular library for making HTTP requests in React applications. It simplifies the process of fetching data from APIs.
Benefits: With Axios, you can easily perform GET, POST, PUT, and DELETE requests. It provides features like request cancellation and interceptors.
5. ESLint and Prettier:
Description: ESLint is a linter tool, while Prettier is a code formatter. Both tools help ensure your code adheres to best practices and consistent styling.
Benefits: Clean, well-formatted code is easier to maintain and collaborate on. These tools catch errors and enforce coding standards.
6. Storybook:
Description: Storybook is an environment for developing and testing UI components in isolation. It's especially useful for component libraries.
Benefits: With Storybook, you can create a visual documentation of your components and test them in isolation, ensuring they work correctly in different scenarios.
7. Jest and Testing Library:
Description: Jest is a JavaScript testing framework, and Testing Library is a set of utilities for testing user interfaces.
Benefits: Writing tests is crucial for ensuring your application works as expected. Jest and Testing Library simplify the process of writing and running tests.
8. DevTools Extensions:
Description: Browser extensions like React DevTools and Redux DevTools provide insights into your application's state and component hierarchy.
Benefits: These tools help you debug, inspect, and profile your React applications, making it easier to identify and fix issues.
📚 The Expansive Ecosystem 📚
React's ecosystem is vast, with countless libraries, tools, and resources available to developers. Whether you need to integrate with GraphQL, manage form state, implement internationalization, or perform animations, there's likely a library that can help. React's large and active community ensures that innovative solutions are always emerging.
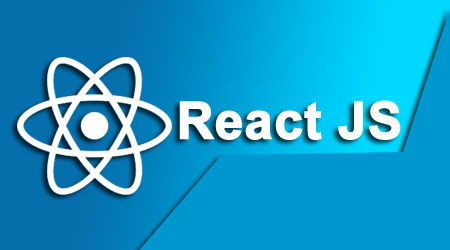
🚀 Mastering React Best Practices 🚀
React development, while empowering, can become complex when building large-scale applications. To ensure the scalability, maintainability, and performance of your React projects, it's essential to follow best practices. In this section, we'll explore some key principles and techniques that will elevate your React development skills.
1. Component-Based Architecture:
Description: React is all about components. Embrace a component-based architecture, breaking down your UI into small, reusable components.
Benefits: This approach simplifies development, promotes reusability, and enhances collaboration among team members.
2. State Management:
Description: For managing application state, choose appropriate tools or libraries like React's built-in state management, Context API, or Redux.
Benefits: Effective state management streamlines data flow and ensures consistency across your app.
3. Props and Prop Types:
Description: Define and document component props using PropTypes. Ensure that components receive the correct data.
Benefits: Prop Types serve as documentation and help catch bugs early during development.
4. Controlled Components:
Description: Implement controlled components for form elements, where React manages the form state.
Benefits: Controlled components provide better control over form inputs and simplify data handling.
5. Immutability:
Description: Avoid direct state mutations. Create copies of objects and arrays to update state.
Benefits: Immutability prevents unintended side effects and makes it easier to track state changes.
6. Component Lifecycle:
Description: Understand the component lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount.
Benefits: Utilize these methods to manage side effects, perform API calls, and clean up resources.
7. Keys for List Rendering:
Description: When rendering lists, provide a unique key prop to each item to help React efficiently update the DOM.
Benefits: Proper keys enable React to recognize and update only the changed items in a list.
8. Performance Optimization:
Description: Optimize your components for better performance using techniques like memoization, PureComponent, and shouldComponentUpdate.
Benefits: Performance optimization ensures smooth user experiences, especially in complex applications.
9. Error Handling:
Description: Implement error boundaries to capture and handle errors gracefully without crashing your entire application.
Benefits: Error boundaries improve application robustness and user experience.
10. Accessibility (a11y): - Description: Prioritize accessibility by using semantic HTML elements and providing proper aria attributes. - Benefits: Accessible applications are inclusive and can be used by a wider audience.
11. Code Splitting: - Description: Utilize code splitting techniques to break your application into smaller bundles that load on-demand. - Benefits: Code splitting improves initial load times and reduces the bundle size.
In summary, React is a powerful JavaScript library for building dynamic user interfaces. In this guide, we covered the fundamentals, core concepts, and best practices for using React. Whether you're a beginner or an experienced developer, React's versatility and ever-evolving ecosystem offer exciting opportunities for web development.
By mastering React, you're well-prepared to create interactive and responsive web applications that captivate users. As you continue your journey, delve into advanced concepts like hooks, context, and Redux for effective state management, and explore techniques for error handling, testing, and performance optimization. With React in your toolkit, you're ready to embark on a world of innovative web development. Happy coding! 🚀
Recent Posts
See AllHey everyone! I hope you're all doing well, constantly evolving, and staying curious in this vast universe we call life. As we all know,...
Comments